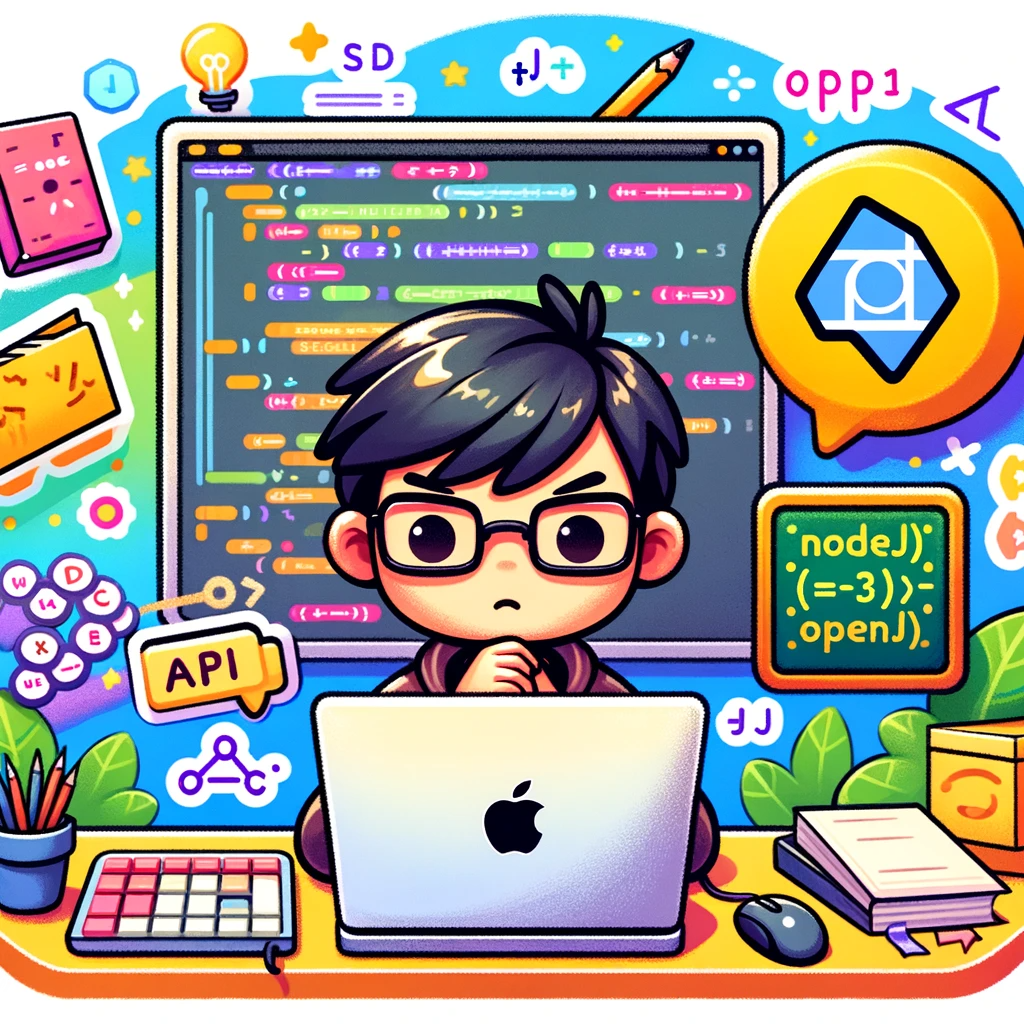
OpenAI 发布了新的助手 API,这为我们提供了许多新的机会。我在发布当天整理了这个简易的 NodeJS 教程,希望能帮助人们更快地开始使用。
- NodeJS*
- OpenAI npm 4.16.1 或更高版本(yarn add openai)
- Readline npm(yarn add readline)
- dotenv npm(yarn dotenv)
- 一个 OpenAPI 密钥
开始编程 创建一个文件并命名为 “.env”,然后在其中添加以下行。不要忘记用您的 OpenAI API 密钥替换文本,您可以从您的 OpenAI 开发者账户中获取这个密钥。
OPENAI_API_KEY="REPLACE WITH YOUR OPENAI API KEY HERE"
接下来,创建另一个文件并命名它为例如 mathTeacher.js。名称并不真正重要,这只是您稍后将从终端运行的文件名。将以下代码粘贴进去。
// import the required dependencies
require("dotenv").config();
const OpenAI = require("openai");
const readline = require("readline").createInterface({
input: process.stdin,
output: process.stdout,
});
// Create a OpenAI connection
const secretKey = process.env.OPENAI_API_KEY;
const openai = new OpenAI({
apiKey: secretKey,
});
async function askQuestion(question) {
return new Promise((resolve, reject) => {
readline.question(question, (answer) => {
resolve(answer);
});
});
}
async function main() {
try {
const assistant = await openai.beta.assistants.create({
name: "Math Tutor",
instructions:
"You are a personal math tutor. Write and run code to answer math questions.",
tools: [{ type: "code_interpreter" }],
model: "gpt-4-1106-preview",
});
// Log the first greeting
console.log(
"\nHello there, I'm your personal math tutor. Ask some complicated questions.\n"
);
// Create a thread
const thread = await openai.beta.threads.create();
// Use keepAsking as state for keep asking questions
let keepAsking = true;
while (keepAsking) {
const userQuestion = await askQuestion("\nWhat is your question? ");
// Pass in the user question into the existing thread
await openai.beta.threads.messages.create(thread.id, {
role: "user",
content: userQuestion,
});
// Use runs to wait for the assistant response and then retrieve it
const run = await openai.beta.threads.runs.create(thread.id, {
assistant_id: assistant.id,
});
let runStatus = await openai.beta.threads.runs.retrieve(
thread.id,
run.id
);
// Polling mechanism to see if runStatus is completed
// This should be made more robust.
while (runStatus.status !== "completed") {
await new Promise((resolve) => setTimeout(resolve, 2000));
runStatus = await openai.beta.threads.runs.retrieve(thread.id, run.id);
}
// Get the last assistant message from the messages array
const messages = await openai.beta.threads.messages.list(thread.id);
// Find the last message for the current run
const lastMessageForRun = messages.data
.filter(
(message) => message.run_id === run.id && message.role === "assistant"
)
.pop();
// If an assistant message is found, console.log() it
if (lastMessageForRun) {
console.log(`${lastMessageForRun.content[0].text.value} \n`);
}
// Then ask if the user wants to ask another question and update keepAsking state
const continueAsking = await askQuestion(
"Do you want to ask another question? (yes/no) "
);
keepAsking = continueAsking.toLowerCase() === "yes";
// If the keepAsking state is falsy show an ending message
if (!keepAsking) {
console.log("Alrighty then, I hope you learned something!\n");
}
}
// close the readline
readline.close();
} catch (error) {
console.error(error);
}
}
// Call the main function
main();
这段代码是在很短的时间内草率地拼凑起来的,还有很多改进的空间。在轮询机制中肯定存在缺陷,但希望这能成为您可以在其基础上构建的起点。
我建议您阅读 OpenAI 助手文档,以了解代码的作用。例如,您只需要创建一次助手,然后可以根据需要通过创建新的线程来调用该助手。我将在未来的视频中更详细地解释这一点。
运行您的代码 要运行您的代码,请打开终端并导航到您保存文件的文件夹。从那里运行 mathTeacher.js 文件。
node mathTeacher.js
声明:本站所有文章,如无特殊说明或标注,均为本站原创发布。任何个人或组织,在未征得本站同意时,禁止复制、盗用、采集、发布本站内容到任何网站、书籍等各类媒体平台。如若本站内容侵犯了原著者的合法权益,可联系我们进行处理。